AOP : Aspect Oriented Programming
AOP가 필요한 상황
- 메소드의 호출 시간을 측정하고 싶을 때
- 회원가입 시간, 회원조회 시간을 측정하고 싶을 때
- 공통 관심 사항(cross-cutting concern) vs 핵심 관심 사항(core concern)
package hello.hellospring.aop;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.springframework.stereotype.Component;
@Component
@Aspect
public class TimeTraceAop {
@Around("execution(* hello.hellospring..*(..))")
public Object execute(ProceedingJoinPoint joinPoint) throws Throwable {
long start = System.currentTimeMillis();
System.out.println("START: " + joinPoint.toString());
try {
return joinPoint.proceed();
} finally {
long finish = System.currentTimeMillis();
long timeMs = finish - start;
System.out.println("END: " + joinPoint.toString()+ " " + timeMs +
"ms");
}
}
}
@Aspect : 타겟 메서드를 감싸서 특정 Advice를 실행
@Around("execution(* hello.hellospring..*(..))") : 해당 경로의 메서드에 이 Aspect를 적용
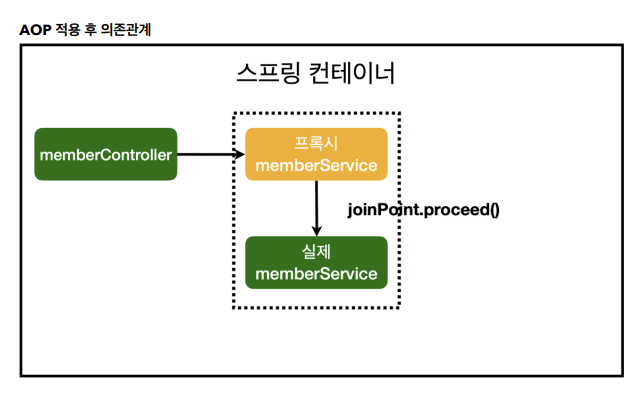
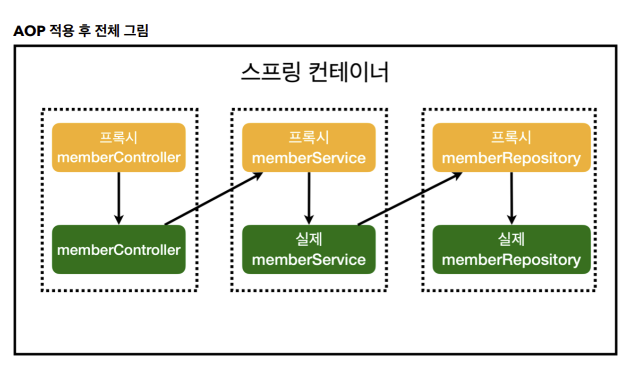
'JAVA' 카테고리의 다른 글
[Spring Boot] p6spy - 외부 라이브러리 사용하기 (1) | 2023.10.25 |
---|---|
[Spring boot] 라이브러리 (1) | 2023.10.25 |
[Spring] @SpringBootTest, @Transactional (0) | 2023.10.25 |
[Spring] jdbc.datasource.DataSourceUtils가 import 되지 않는 오류 (0) | 2023.10.25 |
[Spring] 스프링 빈과 의존관계 (0) | 2023.10.25 |